Python <-> Rust Code Snippets Comparison
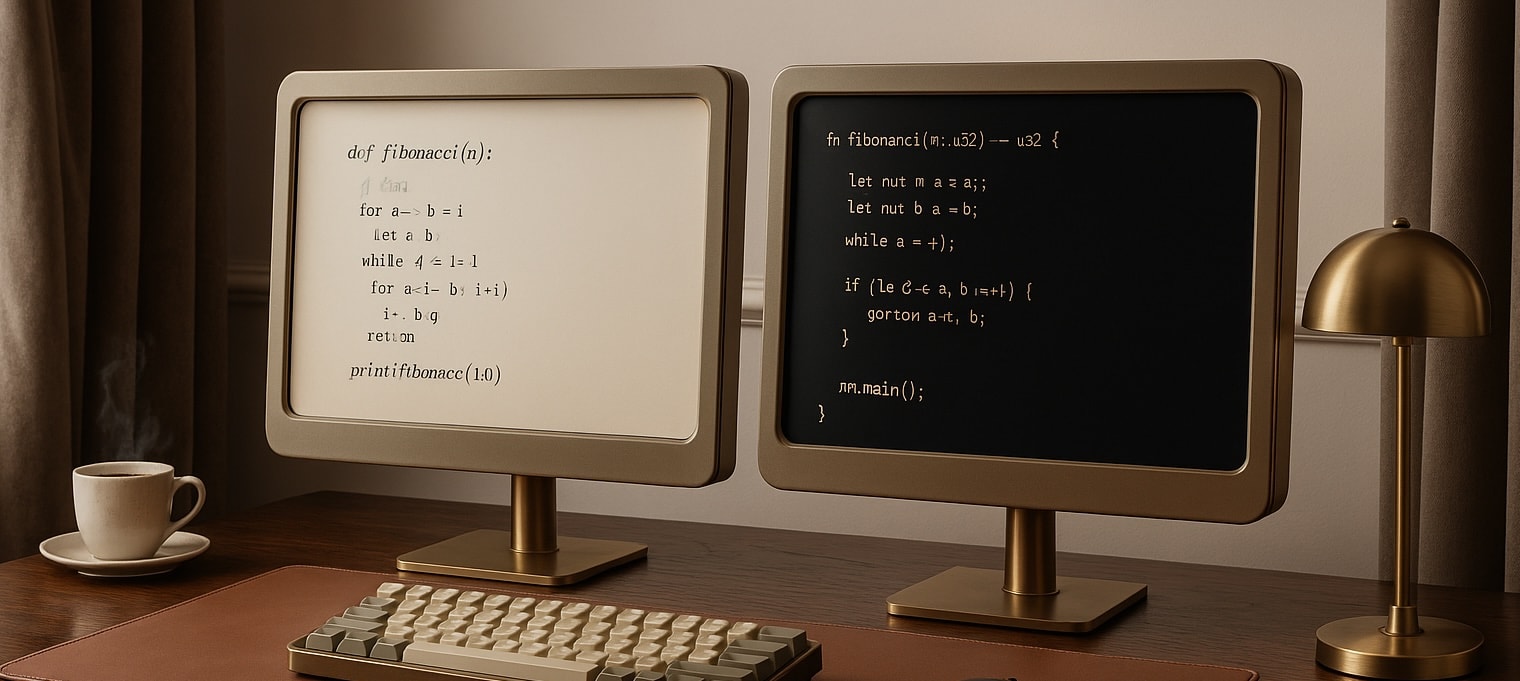
Iteration
Iterating Over Elements
nums = [1, 2, 3, 4, 5]
for x in nums:
print(x)
fn main() {
let nums = vec![1, 2, 3, 4, 5];
for x in &nums {
println!("{}", x);
}
}
Iterating Over Elements with Indexes
nums = [1, 2, 3, 4, 5]
for i, x in enumerate(nums):
print(f"index: {i}, value: {x}")
fn main() {
let nums = vec![1, 2, 3, 4, 5];
for (i, x) in nums.iter().enumerate() {
println!("index: {}, value: {}", i, x);
}
}
Data Structures
Lists and Vectors
nums = [1, 2, 3, 4, 5]
nums.append(6)
fn main() {
let mut nums = vec![1, 2, 3, 4, 5];
nums.push(6);
}
Dictionaries and HashMaps
ages = {'Alice': 30, 'Bob': 25}
ages['Charlie'] = 35
use std::collections::HashMap;
fn main() {
let mut ages = HashMap::new();
ages.insert("Alice", 30);
ages.insert("Bob", 25);
ages.insert("Charlie", 35);
}
Arithmetic Manipulations
a = 10
b = 5
sum = a + b
difference = a - b
product = a * b
quotient = a / b
fn main() {
let a = 10;
let b = 5;
let sum = a + b;
let difference = a - b;
let product = a * b;
let quotient = a / b;
}
remainder = a % b
power = a ** b
fn main() {
let a = 10;
let b = 5;
let remainder = a % b;
let power = a.pow(b as u32); // Note: `pow` requires the exponent to be `u32`
}
Encapsulation
Python (Class-Based)
class Rectangle:
def __init__(self, width, height):
self._width = width # Private attribute
self._height = height
def area(self):
return self._width * self._height
rect = Rectangle(10, 20)
print(rect.area())
Rust (Structs and Methods)
In Rust, encapsulation is achieved using structs and methods. Fields are private by default, and you can define public methods to access them
struct Rectangle {
width: u32,
height: u32,
}
impl Rectangle {
fn new(width: u32, height: u32) -> Self {
Self { width, height }
}
fn area(&self) -> u32 {
self.width * self.height
}
}
fn main() {
let rect = Rectangle::new(10, 20);
println!("Area: {}", rect.area());
}
Inheritance
Python (Class-Based)
Python supports inheritance, allowing you to create subclasses that inherit attributes and methods from a parent class
class Shape:
def area(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
Rust (Traits and Composition)
Rust does not support inheritance. Instead, it uses traits and composition to achieve similar functionality
trait Shape {
fn area(&self) -> f64;
}
struct Rectangle {
width: f64,
height: f64,
}
impl Shape for Rectangle {
fn area(&self) -> f64 {
self.width * self.height
}
}
Polymorphism
Python (Class-Based)
Polymorphism in Python is achieved through inheritance and method overriding
class Shape:
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
shapes = [Circle(5), Rectangle(10, 20)]
for shape in shapes:
print(shape.area())
Rust (Traits and Dynamic Dispatch)
Rust uses traits to achieve polymorphism. You can use trait objects for dynamic dispatch.
trait Shape {
fn area(&self) -> f64;
}
struct Circle {
radius: f64,
}
impl Shape for Circle {
fn area(&self) -> f64 {
std::f64::consts::PI * self.radius * self.radius
}
}
fn print_area(shape: &dyn Shape) {
println!("Area: {}", shape.area());
}
fn main() {
let circle = Circle { radius: 5.0 };
let rect = Rectangle { width: 10.0, height: 20.0 };
print_area(&circle);
print_area(&rect);
}